Search This Blog
Hello there, warm Welcome :-) I am trying to share the knowledge and cool things I learnt during my QA journey!! I hope you find something interesting here :-)
Featured
- Get link
- X
- Other Apps
Useful JavaScript methods for handling arrays in API Testing using Postman!!
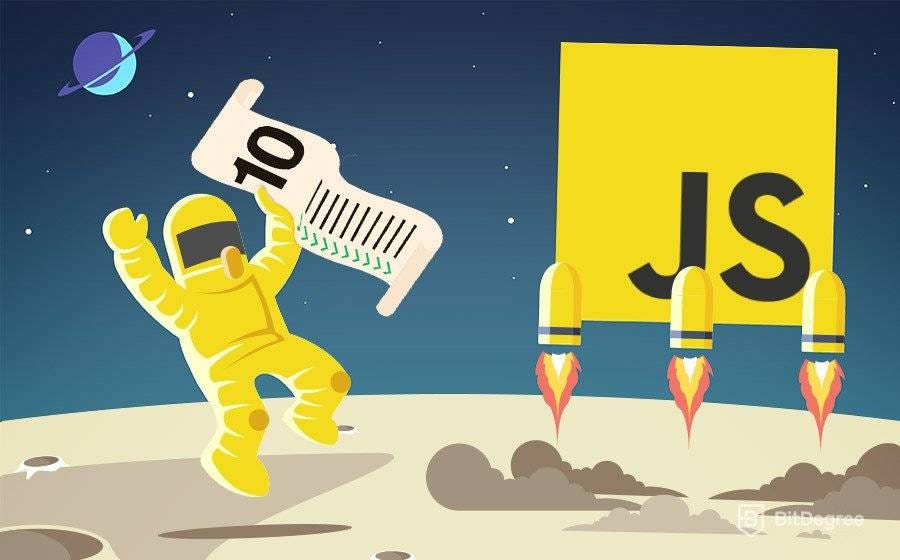
When you are Testing your API, most of the times you need handle JSON Arrays . Based on my experience I would say, some basic array functions would help you to write cool tests in Postman. Nothing to be scared of, it’s actually fun.
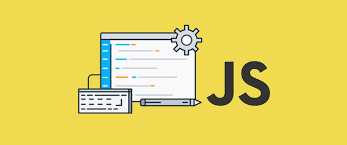
Let’s see the basic and useful functions,
Before that, let’s first define an array for using in our examples.
let names = [‘George’, ‘Annie’, ’Sofia’, ‘Paul’]
And you can directly print the names using console.log(“Person Names: “, names);
To get the property of the array,
Length: This returns the entire length of an array.
Syntax: arrayname.length
Example: console.log(names.length) // gives you the output 4
Below are some common methods used:
1.Push: This method adds the list of values to the end of the array.
Example: names.push(‘Henry’, ‘Adriana’);
console.log(names) // names = [‘George’, ‘Annie’, ’Sofia’, Paul’, ‘Henry’, ‘Adriana’]
2. Unshift: This method adds comma-separated list of values to the front of the array.
Example: names.unshift(‘Nick’, ‘Rio’);
console.log(names) // names = [‘Nick’, ‘Rio’, ‘George’, ‘Annie’, ’Sofia’, Paul’]
3. Pop: This method removes the last value of the array.
Example: names.pop();
console.log(names) // names = [‘George’, ‘Annie’, ’Sofia’]
4. Shift: This method removes the first value of the array.
Example: names.shift();
console.log(names) // names = [‘Annie’, ’Sofia’, ‘Paul’]
5. Splice: This method finds the specified position (pos) and removes n number of items from the array. Arguments are passed in here.
Example: names.splice(3, 1, ‘Ryan’);
// replaces 1 element at index 3
console.log(names);
// names = [‘George’, ‘Annie’, ’Sofia’, ‘Ryan’]
6. Slice: This method creates a copy of an array with selected elements.
Example: var newNames = names.slice(1, 3);
console.log(“New Names: “, newNames );
//newNames = [ ‘Annie’, ’Sofia’]
7. Indexof: This method returns the first element that matches the search parameter after the specified index position. Defaults to index 0.
Example: var result = names.indexOf(‘Sofia’);
console.log(“The search result index is: “, result); // The search result index is: 2
console.log(names.indexOf(‘Mark’)); // -1 (since the value is not present inside the array)
8. Join: This method returns the items in an array as a comma separated string. The separator argument can be used to change the comma to something else.
Example: var arrayString = names.join(‘-’);
console.log(“Strings present in the array: “, arrayString);
//Strings present in the array: George-Annie-Sofia-Paul
console.log(names.join()); // George, Annie, Sofia, Paul
9. Reverse: Literally, it’s the same as the name implies. This method will reverse the array for you.
Example: names.reverse();
console.log(names) //names = [‘Paul’, ‘Sofia’ ,’Annie’ ,’George’]
10. Sort: This method sorts the element of an array, and sorted array is returned.
Example: names.sort();
console.log(names) //names = [‘Annie’, ‘George’, ‘Paul’, ‘Sofia’]
Isn’t real fun?? Hope it will be helpful for writing your automated scripts for API using Postman. You can learn JS, even you are from QA background quickly! All you need is to have a passion and invest some quality time!!!
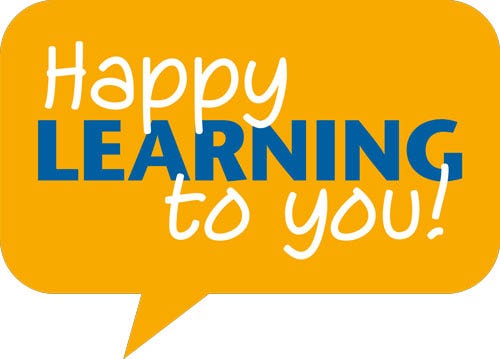
Popular Posts
How to handle SOAP API and parsing the response in Postman??
- Get link
- X
- Other Apps
Comments
Post a Comment