Search This Blog
Hello there, warm Welcome :-) I am trying to share the knowledge and cool things I learnt during my QA journey!! I hope you find something interesting here :-)
Featured
- Get link
- X
- Other Apps
Everything you need to know about JavaScript!!
When I started learning JS, I used many sources.
And of course I have written few articles on JS functions and methods. So I thought of clubbing the simple tricks and basics for JS in single place.
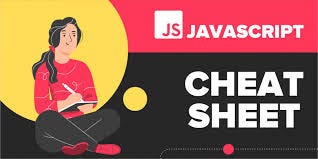
Lets start with the variables.
1.Variables:
var, const, let
var: The most common variable. Can be reassigned but only accessed within a function. Variables defined with var move to the top when code is executed.
const: Cannot be reassigned and not accessible before they appear within the code.
let: Similar to const, however, the let variable can be reassigned but not re-declared.
2. Array Methods:
- concat(): Join several arrays into one
- indexOf(): Returns the first position at which a given element appears in an array
- join(): Combine elements of an array into a single string and return the string
- lastIndexOf(): Gives the last position at which a given element appears in an array
- pop(): Removes the last element of an array
- push(): Adds a new element at the end
- reverse(): Reverse the order of the elements in an array
- shift(): Remove the first element of an array
- slice(): Pulls a copy of a portion of an array into a new array
- sort(): Sorts elements alphabetically
- splice(): Adds elements in a specified way and position
- toString(): Converts elements to strings
- unshift(): Adds a new element to the beginning
- valueOf(): Returns the primitive value of the specified object
3. Operators:
Basic Operators
Addition
- — Subtraction
- Multiplication
- / Division
- (..) Grouping operator
- % Modulus (remainder)
- ++ Increment numbers
- — Decrement numbers
Comparison Operators
- == Equal to
- — Decrement numbers
- === Equal value and equal type
- != Not equal
- !== Not equal value or not equal type
- > Greater than
- < Less than
- >= Greater than or equal to
- <= Less than or equal to
- ? Ternary operator
Logical Operators
- && Logical and ||
- Logical or !
- Logical not
Bitwise Operators
- & AND statement
- OR statement
- ~ NOT
- ^ XOR
- << Left shift
- >>Right shift
- >>> Zero fill right shift
4. Functions:
function name(parameter1, parameter2, parameter3) { // what the function does }
Outputting Data
- alert(): Output data in an alert box in the browser window
- confirm(): Opens up a yes/no dialog and returns true/false depending on user click
- console.log(): Writes information to the browser console, good for debugging purposes
- document.write():Write directly to the HTML document
- prompt(): Creates an dialogue for user input
Global Functions
- decodeURI(): Decodes a Uniform Resource Identifier (URI) created by encodeURI or similar
- decodeURIComponent(): Decodes a URI component
- encodeURI(): Encodes a URI into UTF-8
- encodeURIComponent(): Same but for URI components
- eval(): Evaluates JavaScript code represented as a string
- isFinite(): Determines whether a passed value is a finite number
- isNaN(): Determines whether a value is NaN or not
- Number(): Returns a number converted from its argument
- parseFloat(): Parses an argument and returns a floating point number
- parseInt(): Parses its argument and returns an integer
5. Loops:
for (before loop; condition for loop; execute after loop) { // what to do during the loop }
- for: The most common way to create a loop in JavaScript
- while: Sets up conditions under which a loop executes
- do while: Similar to the while loop, however, it executes at least once and performs a check at the end to see if the condition is met to execute again
- break: Used to stop and exit the cycle at certain conditions
- continue: Skip parts of the cycle if certain conditions are met
6. If — Else Statements:
if (condition) { // what to do if condition is met } else { // what to do if condition is not met }
7. Strings:
var fruit = “Apple”;
Escape Characters
- \’ — Single quote
- \” — Double quote
- \\ — Backslash
- \b — Backspace
- \f — Form feed
- \n — New line
- \r — Carriage return
- \t — Horizontal tabulator
- \v — Vertical tabulator
String Methods
- charAt(): Returns a character at a specified position inside a string
- charCodeAt(): Gives you the unicode of character at that position
- concat(): Concatenates (joins) two or more strings into one
- fromCharCode(): Returns a string created from the specified sequence of UTF-16 code units
- indexOf(): Provides the position of the first occurrence of a specified text within a string
- lastIndexOf(): Same as indexOf() but with the last occurrence, searching backwards
- match(): Retrieves the matches of a string against a search pattern
- replace(): Find and replace specific text in a string
- search(): Executes a search for a matching text and returns its position
- slice():Extracts a section of a string and returns it as a new string
- split(): Splits a string object into an array of strings at a specified position
- substr(): Similar to slice() but extracts a substring depended on a specified number of characters
- substring(): Also similar to slice() but can’t accept negative indices
- toLowerCase(): Convert strings to lowercase
- toUpperCase(): Convert strings to uppercase
- valueOf(): Returns the primitive value (that has no properties or methods) of a string object
8. Regular Expressions:
Pattern Modifiers
e — Evaluate replacement
i — Perform case-insensitive matching
g — Perform global matching
m — Perform multiple line matching
s — Treat strings as single line
x — Allow comments and whitespace in pattern
U — Non Greedy pattern
Brackets
[abc] Find any of the characters between the brackets
[^abc] Find any character not in the brackets
[0–9] Used to find any digit from 0 to 9
[A-z] Find any character from uppercase A to lowercase z
(a|b|c) Find any of the alternatives separated with |
Metacharacters
. — Find a single character, except newline or line terminator
\w — Word character
\W — Non-word character
\d — A digit
\D — A non-digit character
\s — Whitespace character
\S — Non-whitespace character
\b — Find a match at the beginning/end of a word
\B — A match not at the beginning/end of a word
\0 — NUL character
\n — A new line character
\f — Form feed character
\r — Carriage return character
\t — Tab character
\v — Vertical tab character
\xxx — The character specified by an octal number xx
\xdd — Character specified by a hexadecimal number dd
\uxxxx — The Unicode character specified by a hexadecimal number xxxx
Quantifiers
n+ — Matches any string that contains at least one n
n* — Any string that contains zero or more occurrences of n
n? — A string that contains zero or one occurrences of n
n{X} — String that contains a sequence of X n’s
n{X,Y} — Strings that contains a sequence of X to Y n’s
n{X,} — Matches any string that contains a sequence of at least X n’s
n$ — Any string with n at the end of it
^n — String with n at the beginning of it
?=n — Any string that is followed by a specific string n
?!n — String that is not followed by a specific string n
9. Numbers and Math:
Number Methods
- toExponential(): Returns a string with a rounded number written as exponential notation
- toFixed(): Returns the string of a number with a specified number of decimals
- toPrecision(): String of a number written with a specified length
- toString(): Returns a number as a string
- valueOf(): Returns a number as a number
Math Methods
- abs(x) Returns the absolute (positive) value of x
- acos(x) The arccosine of x, in radians
- asin(x) Arcsine of x, in radians
- atan(x) The arctangent of x as a numeric value
- atan2(y,x) Arctangent of the quotient of its arguments
- ceil(x) Value of x rounded up to its nearest integer
- cos(x) The cosine of x (x is in radians)
- exp(x) Value of Ex
- floor(x) The value of x rounded down to its nearest integer
- log(x) The natural logarithm (base E) of x
- max(x,y,z,…,n) Returns the number with the highest value
- min(x,y,z,…,n) Same for the number with the lowest value
- pow(x,y) X to the power of y
- random() Returns a random number between 0 and 1
- round(x) The value of x rounded to its nearest integer
- sin(x) The sine of x (x is in radians)
- sqrt(x) Square root of x
- tan(x) The tangent of an angle
I hope it will be useful to you all guys!!
Popular Posts
How to handle SOAP API and parsing the response in Postman??
- Get link
- X
- Other Apps
Comments
Post a Comment